+
Ask a programmer how a beginner should automate web tasks and chances are good they’ll say Python.
Python is a programming language that can help you automate everything from email sends to web scraping to robotics, and while it’s significantly more complicated than starting off with UBot Studio, it’s a very robust language and fairly easy to understand for beginners. (For more info on getting started with Python, click here to read about it and here to get it set up on your computer.)
Because at times using Python will make your bots faster, smarter, and more awesome, we included Python integrations inside of UBot Studio 5.5. As a Standard or Professional user, you will be able to run IronPython scripts. As a Developer user, you’ll be able to write and edit Python scripts directly inside UBot.
Seth and the Team had a great conversation with the UBot Studio community about how you can get set up using Python, what you might want to do with it to start, and the way that Python integration also allows you to add .dll files to your bots. There will be more Python conversations in the future and we’ll be sure to tie all these chats together on the blog!
For more info, check out the Run_Python command on our wiki.
Community Chat: Getting Started with Python
Seth Turin:
Python is a great language for beginning programmers and expert programmers alike. I chose Python because it’s widely regarded as the easiest language to learn, while still being a fully expressive, object oriented language.
Jason Kelley:
Yeah – I’m barely a programmer and even I’ve used it a little to do some really cool stuff, after getting some scripts from some other programmers and editing them.
Seth:
To get started into Python with UBot, you’ll need to have IronPython installed on your system. We’re working on removing this as a dependency, but for now, you can download and install IronPython at http://IronPython.codeplex.com/releases/view/90087
Jason:
And IronPython is a type of Python? In the way that Mysql is a type of SQL?
Dave: 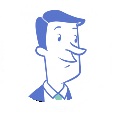
IronPython works with .net, I think.
Seth:
Yes, IronPython is an implementation of the Python spec that is built on the .net framework. It’s a nice implementation, too, because you not only get all of Python’s yumminess, you also get the entire .net framework! Once you have IronPython installed, you should be able to use Python, its core modules, and the Python Standard Library.
Let’s do a few examples to demonstrate how to have fun with IronPython in UBot.
alert($run python with result("import datetime mydate = datetime.date(1943,3, 13) #year, month, day mydate.strftime("%A")"))
What I’m doing with this bit of code is making a Python function return a result back, that I can show in an alert, or of course, do anything I want with. UBot’s date functions are somewhat limited, so just having a few lines of Python handy can give you a lot of power. The %A in the last line indicates day of the week. If you want to see more tricks with the datetime module, goes here http://www.Pythonforbeginners.com/basics/Python-datetime-time-examples
The Python standard library has a lot of stuff! This opens up UBot’s capabilities in a huge way. A full reference on the standard library is here https://docs.Python.org/2/library/
So what if we wanted to take our date example earlier and make it a custom function?
alert($get that date()) define $get that date { return($run python with result("import datetime mydate = datetime.date(1943,3, 13) #year, month, day mydate.strftime("%A")"))}
If you consider the possibilities here, you could easily make an entire bot of just defines, like a library, and include it into all your other bots. This effectively gives you the same power as creating plugins, though plugins have a few differences.
Jason:
Does using Python change the size of your bots etc. now that you have to use the IronPython framework?
Seth:
Yes, but not significantly. About as much as a plugin would and maybe less.
Seth:
So, there’s more that you’ll want to know about how IronPython works with .net because it gives you a lot of great new options. IronPython comes equipped with a few special commands for importing .net framework libraries. Let me show you an example:
import clr clr.AddReference('System.Speech') from System.Speech.Synthesis import SpeechSynthesizer speechSynthesizer = SpeechSynthesizer() speechSynthesizer.SpeakAsync("hello I am bot")
A few things happen here:
1) We import clr. That’s basically where the core .net stuff lives.
2) We add a reference to system.speech, which is part of the .net framework.
3) Using some Python syntax, we get our speech synthesizer fired up
4) We tell it to talk in awkward English.
Jason:
Whoa, does this make my bot talk to me?
Seth:
Yes. Your bot now has a voice and it just wants to be heard.
Another implication of the .net framework is that you can load .net dll files. So any .net open source project that you want to utilize can now be a part of your bot. Here’s an example:
import clr clr.AddReferenceToFileAndPath(UBot.VerifyLibrary("NAudio.dll")) import sys import NAudio from System.IO import File waveOutDevice = NAudio.Wave.WaveOut() audioFileReader = NAudio.Wave.AudioFileReader("c:\sounds\sound.mp3") waveOutDevice.Init(audioFileReader) waveOutDevice.Play()
I start by importing clr again, but then I use AddReferenceToFileAndPath to load the dll. In this script, I use a special function that UBot has loaded to help manage libraries for use in the bot bank. To load your own dll, it might look more like:
clr.AddReferenceToFileAndPath(“NAudio.dll”) or clr.AddReferenceToFileAndPath(“c:\lib\NAudio.dll”)
Note that path names have double backslashes. Once I load up the dll, I import the stuff I want from it, along with some core modules for loading files, and I have my wave player play a sound file. For clarity’s sake, I’ve just been pasting the Python code that you would paste into a run Python command. The full uscript for this example looks like this:
run python("import clr clr.AddReferenceToFileAndPath(UBot.VerifyLibrary("NAudio.dll")) import sys import NAudio from System.IO import File waveOutDevice = NAudio.Wave.WaveOut() audioFileReader = NAudio.Wave.AudioFileReader("c:\\sounds\\sound.mp3") waveOutDevice.Init(audioFileReader) waveOutDevice.Play()")
Jason:
So I’m using Python to include .net stuff and now my bot is essentially capable of the same things that a .net script is (or at least a lot of those things)?
Seth:
IronPython is .net, so everything, yes
jay_ar233:
So IronPython is like gateway for Python to run on .net?
Seth:
That’s one way to look at it Jay.
Jason:
Ok, so I think the only thing left that’s confusing to me is how my Python script is interacting with .net dll’s – because aren’t they written in .net. Like I’m using Python commands to reference .net commands which is a different language?
Seth:
Good question, the important thing there is that .net itself isn’t a language. .Net is a framework, written probably in C or C++, that a few languages are built on, such as VB.Net, C#, and IronPython. .Net uses a construct called the clr which stands for common language runtime – you saw references in the scripts earlier. C#, VB.Net, and IronPython all compile down to the clr. Make sense? All this is to say, IronPython can read dlls compiled in any of the previously mentioned clr languages.
Jason:
Whoa, does Python completely open UBot up to being able to use all those languages, in a manner of speaking? In the sense that you can write modules in C# or VB, and then import them using 1 or 2 lines of Python?
Seth:
I hadn’t thought of that, but yes.
Some of you may want to know if IronPython or C# is faster. That question did come up while we were working on this, because we’re always wondering how we can make UBot faster and more agile while remaining easy to use. There’s a great discussion of this on stackoverflow:
http://stackoverflow.com/questions/3081661/speed-of-code-execution-IronPython-vs-c
In programming, there’s always a tradeoff. Assembly is the fastest language, by far, but it takes forever to write, and is awful. C++ is still fast, but slightly easier to write, C# is less fast and very easy to write, IronPython is less fast and extremely easy to write. Uscript is easier and slower than all of the previously mentioned languages. The tradeoff is speed of processing vs speed of implementation. The reason that UBot is so valuable is that you can write a UBot bot in 2 hours that does the same thing as a C# bot that took 2 weeks to write, because of all the built-in features of UBot. The C# bot is faster, but if you’re two weeks ahead of your competition, you clearly have the edge. Now I’m getting all theoretical, but the point is we now have all these options.
Jason:
Like any serious programmer should really now be able to build incredible bots for UBot far outside of the uscript framework? And any non-serious programmer can move in that direction if they want?
Seth:
Absolutely.
If you want to dive deeper into the wonderful world of Python, here are some great learning resources.
- If you want to get into some heavier script, I recommend using a full IronPython IDE to write and test your script before pasting it into UBot: http://codecondo.com/10-ways-to-learn-Python/
- Visual studio actually works great for this. You can download visual studio community edition here: http://www.visualstudio.com/products/visual-studio-community-vs.
- Once you have IronPython and visual studio installed, you’ll need Python tools: https://pytools.codeplex.com/downloads/get/920477
- And just a reminder, you can download IronPython here: https://pytools.codeplex.com/downloads/get/920477
Have fun with it! I think I speak for the whole team when I say that I’m eager to see what you guys come up with now with the limitless possibilities that Python provides.